One common task when working with HTML forms is to check whether a checkbox is checked.
This can be done using JavaScript or jQuery.
A popular JavaScript library like jQuery comprises Javascript functions that make it easy for web developers to do common tasks like handling user events, manipulating the webpage, etc.
A checkbox is a type of input element that permits users to select one or multiple options from a list.
When the form is submitted, only the value of the checked checkboxes is sent to the server.
The HTML/ CSS Code used to check whether a checkbox is checked in jQuery
Below is the HTML code that we will use in this post. It contains a heading tag, a checkbox input with an id “myCheckbox”, and a header tag with an id “helloWorld”.
It also includes a reference to an external CSS file named “index.css” for styling.
The line <script src=”https://code.jquery.com/jquery-3.6.3.js”></script> is a reference to an external JavaScript library called jQuery. Check out our comprehensive post and learn about The 2 Best Ways to Add jQuery to HTML.
The line <script src=”index.js”></script> is a reference to a separate JavaScript file named “index.js” that contains the jQuery code that we will use in this post.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
<html> <head> <script src="https://code.jquery.com/jquery-3.6.3.js"></script> <link rel="stylesheet" href="index.css" /> </head> <body bgcolor="skyblue"> <h2>Check Whether a Checkbox Is Checked In Jquery?</h2> <input type="checkbox" id="myCheckbox" /> <h1 id="helloWorld">Hello World</h1> <script src="index.js"></script> </body> </html> |
The CSS code below styles the HTML document, with the following effects:
- The heading element (<h1>) will be colored brown.
- The element with the ID helloWorld will not be displayed on the page.
- The body element will be displayed as a flex container with its child elements centered both horizontally and vertically. It will have a top margin of 10em.
Note: The display: none property set for #helloWorld hides the element from view when the page loads. This post will show you how to make this element visible when the checkbox is checked.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
h1 { color: brown; } #helloWorld { display: none; } body { display: flex; justify-content: center; flex-direction: column; align-items: center; margin-top: 10em; } |
1. How to check whether a checkbox is checked in jQuery using the is() method
The is() method can be used to check if a checkbox is checked or not. This method returns a Boolean value, true if the checkbox is checked, and false if it is not.
The code below shows you how to check whether a checkbox is checked using the is() method in jQuery.
1 2 3 4 5 6 7 8 9 10 11 |
$(document).ready(function () { $("#myCheckbox").change(function () { if ($(this).is(":checked")) { console.log("Checkbox is checked"); $("#helloWorld").show() } else { console.log("Checkbox is not checked"); $("#helloWorld").hide() } }); }); |
Below is a step-by-step explanation of what is happening:
The first line of code $(document).ready(function () { is a jQuery shorthand method that ensures that the Javascript code is executed after the whole HTML document is loaded.
It then attaches an event listener to this element, specifically for the change event. This means that the code inside the function will be executed whenever the checkbox is checked or unchecked.
The code inside the event listener begins by selecting the checkbox element with the ID “myCheckbox” using the jQuery selector: $(“#myCheckbox”).
Inside the function, the code first checks whether the checkbox is checked or not by using the jQuery is() method with the :checked selector. This returns a boolean value (true if checked, false if not).
If the checkbox is checked, the code logs the message “Checkbox is checked” to the console using the console.log() method.
It then selects an element with the ID “helloWorld” using the jQuery selector $(“#helloWorld”) and calls the show() method on it to display it.
If the checkbox is not checked, the code logs the message “Checkbox is not checked” to the console. It then selects the same element with the ID “helloWorld” and calls the hide() method to hide the element.
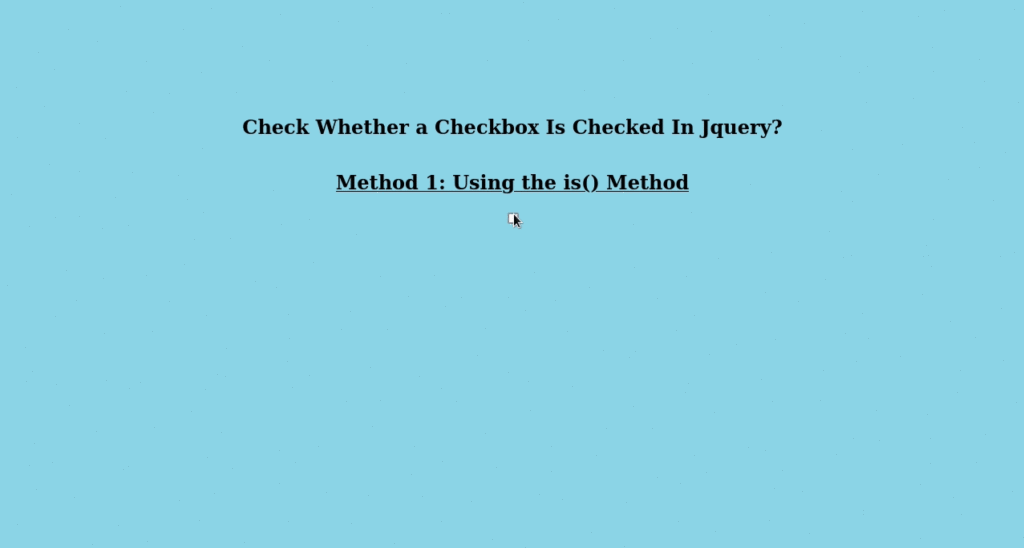
2. How to check whether a checkbox is checked in jQuery using the prop() method
The prop() method can be used to get or set the properties of an element. We can use this method to check if a checkbox is checked by getting the value of the “checked” property.
The code below shows you how to check whether a checkbox is checked using the prop() method in jQuery.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$(document).ready(function () { $("#myCheckbox").change(function () { $(document).ready(function(){ if($('#myCheckbox').prop('checked')){ console.log('Checkbox is checked'); $("#helloWorld").show() } else { console.log('Checkbox is not checked'); $("#helloWorld").hide() } }); }); }); |
Below is a step-by-step explanation of the code above and how it works.
The .change() function in this code is used as an event handler for the change event of the checkbox with the ID “myCheckbox.”
It is triggered every time the state of the checkbox changes, i.e., when it is checked or unchecked by the user.
The code inside the function is executed whenever the checkbox is changed.
The $(document).ready() function ensures that the Javascript code is executed after the whole HTML document is loaded.
When the checkbox with the ID “myCheckbox” is changed, the prop() method is used to check if it is currently checked or not.
If the checkbox is checked, the message “Checkbox is checked” is logged to the console, and a text field with the ID “helloWorld” is shown on the page.
If the checkbox is not checked, the message “Checkbox is not checked” is logged to the console, and the text field is hidden.
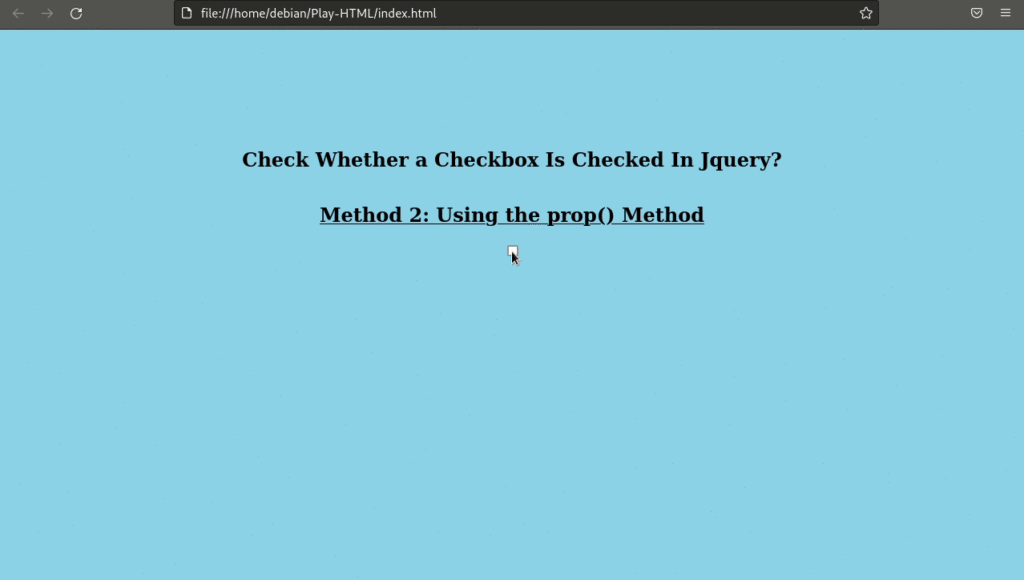
Can I Use the attr() Method to Check Whether a Checkbox Is Checked In jQuery?
Since jQuery 1.6 and other later versions, developers are discouraged from using the attr() to retrieve an element’s checked state.
Prior to version 1.6, the attr() method could be used to get the checked state of a checkbox, but in later versions, it returns the initial value of the attribute and not the current state.
Instead, it’s better to use the prop() method.
Using prop() to check whether a checkbox is checked is simple. You just need to pass the string ‘checked’ to the method, and it will return true if the checkbox is checked or false if it’s not.
See the example below.
1 2 3 4 5 |
if ($('#myCheckbox').prop('checked')) { alert('Checkbox is checked'); } else { alert('Checkbox is not checked'); } |
Can I Use the val() Method to Check Whether a Checkbox Is Checked In jQuery?
No, you cannot use the val() method in jQuery to check whether a checkbox is checked. The val() method is used to get or set the value of form elements such as input, select, and text area.